Path overview
In this path, you’ll develop key technical skills for data scientists, including object-oriented and functional programming with Python, along with libraries like scikit-learn, Matplotlib, NumPy, and pandas. You’ll also learn web scraping, SQL queries, deep learning, machine learning, and predictive analysis. To help you stand out, you’ll explore tools like the UNIX command line, Git, and GitHub for better collaboration.
Best of all, you’ll learn by doing – you’ll write code and get feedback directly in the browser. You’ll apply your skills to several guided projects involving realistic business scenarios to build your portfolio and prepare for your next interview.
Key skills
- Programming with Python to perform complex statistical analysis of large datasets
- Performing SQL queries and web-scraping to explore and extract data from databases and websites
- Building insightful data visualizations to tell stories
- Automating machine learning algorithms and build predictive modeling processes
Path outline
Part 1: Python Introduction [4 courses]
Introduction to Python Programming 3h
Objectives- Write computer programs using Python
- Save values using variables
- Process numerical data and text data
- Create lists using Python
Basic Operators and Data Structures in Python 5h
Objectives- Use for loops to repeat processes and conduct data analysis
- Implement if, else, and elif statements in programming logic
- Employ logical and comparison operators in Python
- Develop and update Python dictionaries for data manipulation
- Construct frequency tables using dictionaries for data analytics
Python Functions and Jupyter Notebook 7h
Objectives- Write Python functions
- Debug functions
- Define function arguments
- Write functions that return multiple variables
- Employ Jupyter notebook
- Build a portfolio project
Intermediate Python for Data Science 8h
Objectives- Clean and analyze text data
- Define object-oriented programming in Python
- Process dates and times
Part 2: Data Analysis and Visualization [3 courses]
Introduction to Pandas and NumPy for Data Analysis 13h
Objectives- Improve your workflow using vectorized operations
- Select data by value using Boolean indexing
- Analyze data using pandas and NumPy
Introduction to Data Visualization in Python 8h
Objectives- Visualize time series data with line plots
- Define correlations and visualize them with scatter plots
- Visualize frequency distributions with bar plots and histograms
- Improve your exploratory data visualization workflow using pandas
- Visualize multiple variables using Seaborn's relational plots
Telling Stories Using Data Visualization and Information Design 5h
Objectives- Create graphs using information design principles
- Create narrative data visualizations using Matplotlib
- Create visual patterns using Gestalt principles
- Control attention using pre-attentive attributes
- Employ Matplotlib's built-in styles
Part 3: Data Cleaning [3 courses]
Data Cleaning and Analysis in Python 11h
Objectives- Employ data aggregation techniques
- Combine datasets
- Transform and reshape data
- Clean strings and resolve missing data
Advanced Data Cleaning in Python 8h
Objectives- Clean and manipulate text data using regular expressions
- Employ lambda functions and list comprehension with pandas
- Resolve missing data
Data Cleaning Project Walkthrough 7h
Objectives- Complete a data cleaning project from start to finish
- Improve your data cleaning skills
Part 4: The Command Line [2 courses]
Command Line for Data Science 4h
Objectives- Employ the command line for data science
- Define important command line concepts
- Modify the behavior of commands with options
- Navigate the filesystem
- Employ glob patterns and wildcards
- Manage users and permissions
Text Processing for Data Science 4h
Objectives- Read and explore documentation
- Inspect files
- Perform basic text processing
- Define different kinds of output
- Redirect and pipe output
- Employ streams and file descriptors
Part 5: Working with Data Sources Using SQL [6 courses]
Introduction to SQL and Databases 5h
Objectives- Define the structure of SQL
- Create basic queries to extract data from tables in a database
- Define databases
- Identify different versions of SQL
- Write good SQL code
Summarizing Data in SQL 3h
Objectives- Employ SQL to compute statistics
- Provide statistics by group
- Filter results over groups
Combining Tables in SQL 3h
Objectives- Combine tables using inner joins
- Employ different types of joins
- Employ other SQL clauses with joins
- Join on complex conditions
- Employ set operators like UNION and EXCEPT
Querying Databases with SQL and Python 1h
Objectives- Connect to a SQLite database using Python
- Query a SQLite database using Python
- Convert data from a SQLite database to a Pandas dataframe
SQL Subqueries 6h
Objectives- Nest a query inside another query
- Employ different types of subqueries
- Employ common table expressions
- Scale your project with complex queries
Window Functions in SQL 7h
Objectives- Set up a frame for window functions
- Compute running aggregations with aggregate window functions
- Explore rank window functions
- Apply distribution window functions
- Use offset window functions
Part 6: APIs and Web Scraping in Python [2 courses]
APIs and Web Scraping in Python for Data Science 5h
Objectives- Understand the basics of APIs, the requests library, and JSON data handling in Python
- Master API authentication, manage API keys, and learn to handle rate limits
- Use optional query parameters for refined data retrieval from APIs
- Develop skills in extracting and analyzing data from web pages
- Integrate API data with Pandas for in-depth data analysis and visualization
Data Analysis for Business in Python 6h
Objectives- Resolve fuzzy language
- Identify the business context of data science
- Communicate with a non-technical audience
- Define metrics
Part 7: Probability and Statistics [5 courses]
Introduction to Statistics in Python 9h
Objectives- Sample data using simple random sampling, stratified sampling, and cluster sampling
- Measure variables in statistics
- Create frequency distribution tables
Intermediate Statistics in Python 8h
Objectives- Summarize a distribution using the mean, the weighted mean, the median, or the mode
- Measure the variability of a distribution using the variance and the standard deviation
- Compare values using z-scores
Introduction to Probability in Python 4h
Objectives- Estimate theoretical and empirical probabilities
- Employ the fundamental rules of probability
- Employ combinations and permutations
Introduction to Conditional Probability in Python 6h
Objectives- Assign probabilities based on conditions
- Assign probabilities based on event independence
- Assign probabilities based on prior knowledge
- Create spam filters using multinomial Naive Bayes
Hypothesis Testing in Python 4h
Objectives- Perform a permutation test
- Perform significance testing to understand an outcome's importance
- Define regular and multi-category chi-squared tests
Part 8: Machine Learning in Python [9 courses]
Introduction to Supervised Machine Learning in Python 8h
Objectives- Establish a machine learning workflow
- Implement the K-Nearest Neighbors algorithm for a classification task from scratch using Pandas
- Implement the K-Nearest Neighbors algorithm using scikit-learn
- Evaluate a machine learning model
- Find optimal hyperparameter values using grid search
Introduction to Unsupervised Machine Learning in Python 6h
Objectives- Identify applications of unsupervised machine learning
- Implement a basic k-means algorithm
- Evaluate and optimize the performance of a k-means model
- Visualize the model
- Build a k-means model using scikit-learn
Calculus For Machine Learning 2h
Objectives- Define mathematical functions using calculus
- Employ intermediate machine learning techniques
Linear Algebra For Machine Learning 3h
Objectives- Define linear systems using linear algebra
- Employ intermediate machine learning techniques
Linear Regression Modeling in Python 4h
Objectives- Describe a linear regression model
- Construct a linear regression model and evaluate it based on the data
- Interpret the results of a linear regression model
- Use a linear regression model for inference and prediction
Gradient Descent Modeling in Python 4h
Objectives- Code a basic Gradient Descent algorithm
- Recognize the limitations of basic Gradient Descent
- Contrast the basic Batch and Stochastic Gradient Descent uses
- Visualize Stochastic Gradient Descent using Matplotlib
- Apply Stochastic Gradient Descent in Python using Scikit Learn
Logistic Regression Modeling in Python 4h
Objectives- Describe a logistic regression model
- Construct a logistic regression model and evaluate it based on the data
- Interpret the results of a logistic regression model
- Use a logistic regression model for inference and prediction
Decision Tree and Random Forest Modeling in Python 6h
Objectives- Create, customize, and visualize decision trees
- Use and interpret decision trees on new data
- Calculate optimal decision paths
- Optimize trees by altering their parameters
- Apply the random forest prediction technique
Optimizing Machine Learning Models in Python 4h
Objectives- Distinguish between different optimization techniques
- Identify the best optimization approach for your project
- Apply optimization methods to improve your model
- Employ machine learning tools on various optimization methods
Part 9: Deep Learning in Python [1 course]
Introduction to Deep Learning in TensorFlow 6h
Objectives- Explain the major concepts and terminology used in deep learning
- Perform data preprocessing and exploratory analysis to prepare data for modeling
- Build and evaluate deep learning regression models using TensorFlow’s Sequential and Functional APIs in Keras
- Build a deep neural network to predict listing gains of IPOs on the Indian market
Part 10: Advanced Topics in Data Science [3 courses]
Intermediate Command Line for Data Science 3h
Objectives- Employ Jupyter console
- Process data from the command line
Introduction to Git and Version Control 3h
Objectives- Organize your code using version control
- Employ Git and GitHub to collaborate with others
- Resolve conflicts in version control
Analyzing Large Datasets in Spark and Map-Reduce 3h
Objectives- Identify the map-reduce framework for breaking down tasks
- Employ Spark to process raw files
- Process structured datasets using Spark SQL and Spark DataFrames
The Dataquest guarantee
Dataquest has helped thousands of people start new careers in data. If you put in the work and follow our path, you’ll master data skills and grow your career.
We believe so strongly in our paths that we offer a full satisfaction guarantee. If you complete a career path on Dataquest and aren’t satisfied with your outcome, we’ll give you a refund.
Master skills faster with Dataquest
Go from zero to job-ready
Learn exactly what you need to achieve your goal. Don’t waste time on unrelated lessons.
Build your project portfolio
Build confidence with our in-depth projects, and show off your data skills.
Challenge yourself with exercises
Work with real data from day one with interactive lessons and hands-on exercises.
Showcase your path certification
Share the evidence of your hard work with your network and potential employers.
Projects in this path
Profitable App Profiles for the App Store and Google Play Markets
For this project, you’ll be a data analyst at a company that builds free, ad-supported Android and iOS apps. To drive revenue, you’ll analyze real app market data to find app profiles that attract the most users.
Exploring Hacker News Posts
For this project, we’ll be data analysts exploring Hacker News posts. We’ll use Python string manipulation, OOP, and date handling to analyze trends driving post popularity. Check out our Jupyter Notebook Guided Project if needed.
Exploring eBay Car Sales Data
For this project, we’ll assume the role of data analysts for a used car classifieds service to explore and clean a dataset of car listings from eBay Kleinanzeigen, a section of the German eBay website.
Finding Heavy Traffic Indicators on I-94
For this project, you’ll assume the role of a data analyst exploring a dataset on westbound traffic on the I-94 Interstate highway. You’ll apply exploratory data visualization techniques to determine indicators of heavy traffic.
Storytelling Data Visualization on Exchange Rates
For this project, we’ll assume the role of a data analyst tasked with creating an explanatory data visualization about Euro exchange rates to inform and engage an audience.
Plus 21 more projects
Learning resources
Grow your career with
Dataquest.
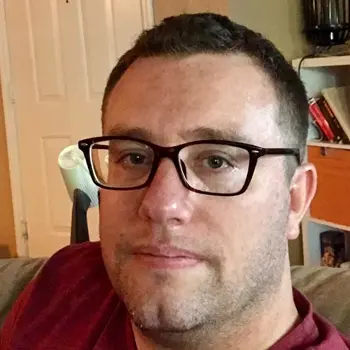
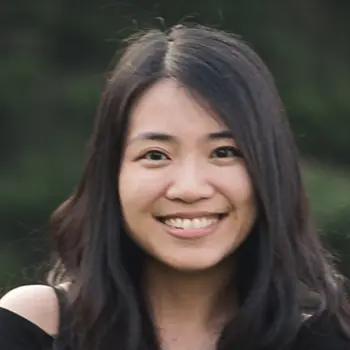
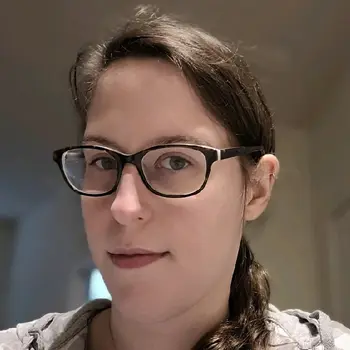