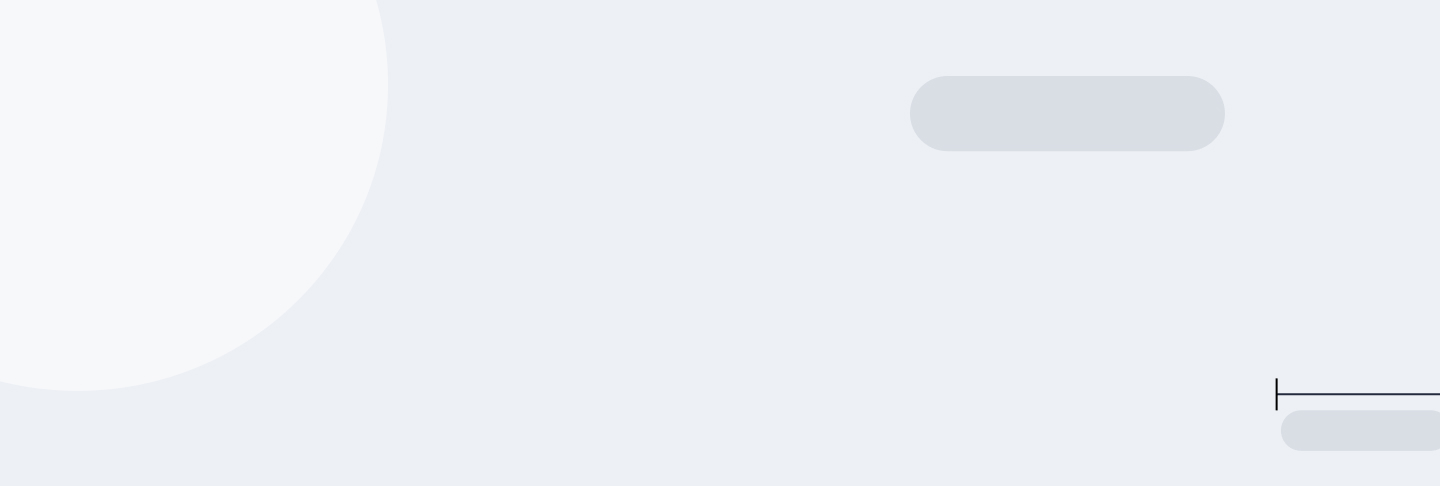
Complete Guide to Python
A collection of Python tutorials, practice problems, cheat sheet, guided projects, and frequently asked questions.
This comprehensive guide, featuring Python tutorials, a cheat sheet, and real-world data science projects, offers everything you need to get started with Python for data science. The included FAQs address common challenges, making it an essential resource for Python beginners.
Python Tutorials
The four Python tutorials summarized below will help support you on your journey to learning Python for data science. Each tutorial is easily accessible with a simple click and is thoughtfully designed to build strong foundational skills. However, If you're just starting out and want to actively learn Python directly in your browser, enroll in Dataquest's Data Scientist in Python skill path for free.
1. Introduction to Python Programming
Introduction to Python Programming — here's a breakdown of what this introduction to Python tutorial teaches:
Lesson 1 – Python Programming
- Learn the concept of programming as giving instructions to a computer
- Understand Python's syntax and how it processes simple arithmetic operations
- Write and execute basic Python code using the
print()
function
Lesson 2 – Python Variables
- Create and use variables to store and manipulate data efficiently
- Understand how variables can be updated and used in calculations
- Apply variables to represent and analyze real-world data
Lesson 3 – Python Data Types
- Differentiate between integers, floats, and strings in Python
- Use the
type()
function to identify data types and avoid calculation errors - Manipulate different data types to perform various analytical tasks
Lesson 4 – Python Lists
- Create and manipulate lists to store multiple items of different data types
- Use indexing to access specific elements within a list
- Apply lists to organize and analyze complex datasets efficiently
2. Basic Operators and Data Structures in Python
Basic Operators and Data Structures in Python — here's a breakdown of what this introduction to Python tutorial teaches:
Lesson 1 – Python For Loops
- Understand the structure and functionality of
for
loops in Python - Use
for
loops to iterate over lists and perform actions on each item - Apply
for
loops to process large datasets efficiently
Lesson 2 – Making Decisions with Python: If, Else, and Elif Statements
- Learn how to use
if
,else
, andelif
statements for conditional execution - Apply comparison operators like
>
,<
,==
, and!=
in conditional statements - Use conditional statements to categorize data based on specific criteria
Lesson 3 – Working with Multiple Conditions in Python
- Combine multiple conditions using logical operators
- Create complex decision trees with nested conditional statements
- Apply multiple conditions to categorize data effectively
Lesson 4 – Organizing Data with Python Dictionaries
- Understand the structure and benefits of Python dictionaries
- Create and manipulate key-value pairs in dictionaries
- Use dictionaries to organize and access complex datasets
Lesson 5 – Creating Frequency Tables with Python Dictionaries
- Utilize dictionaries to create frequency tables from datasets
- Convert raw counts to proportions and percentages
- Analyze data distributions using frequency tables
Lesson 6 – Bringing It All Together
- Combine
for
loops, conditional statements, and dictionaries in data analysis - Create sophisticated data processing workflows
- Apply learned concepts to solve real-world data analysis problems
3. Python Functions and Jupyter Notebook
Python Functions and Jupyter Notebook — here's a breakdown of what this introduction to Python tutorial teaches:
Lesson 1 – Using Built-in Functions and Creating Functions
- Utilize Python's built-in functions like
sum()
,len()
, andmax()
for efficient data analysis - Create custom functions to package repetitive operations and improve code organization
- Understand the concept of function parameters and arguments
Lesson 2 – Arguments, Parameters, and Debugging
- Differentiate between function parameters and arguments for more flexible code
- Use keyword and positional arguments when calling functions
- Implement debugging techniques, such as strategic
print()
statements, to troubleshoot functions
Lesson 3 – Built-in Functions and Multiple Return Statements
- Explore advanced uses of built-in functions for data manipulation
- Implement multiple
return
statements to create more versatile functions - Avoid common pitfalls, such as shadowing built-in functions
Lesson 4 – Returning Multiple Variables and Function Scopes
- Return multiple variables from a single function using tuples
- Understand local and global scopes to manage variable accessibility
- Use tuple unpacking to assign multiple returned values to separate variables
Lesson 5 – Learn and Install Jupyter Notebook
- Install Jupyter Notebook through the Anaconda distribution
- Navigate the Jupyter Notebook interface and execute code in cells
- Utilize magic commands like
%history
and%timeit
for enhanced functionality
Guided Project: Profitable App Profiles for the App Store and Google Play Markets
- Apply Python functions and Jupyter Notebook to analyze real-world app store data
- Create custom functions for data exploration and frequency table generation
- Combine multiple functions to perform complex data analysis tasks efficiently
4. Intermediate Python for Data Science
Intermediate Python for Data Science — here's a breakdown of what this introduction to Python tutorial teaches:
Lesson 1: Cleaning and Preparing Data in Python
- Use the
replace()
method to clean and standardize string data - Create functions to remove multiple unwanted characters from strings
- Implement data cleaning techniques to handle inconsistencies in real-world datasets
Lesson 2: Python Data Analysis Basics
- Develop functions to summarize and explore datasets efficiently
- Utilize string formatting to present analysis results clearly
- Apply basic data analysis techniques to extract insights from datasets
Lesson 3: Object-Oriented Python: A Powerful Approach for Data Science
- Understand the fundamentals of object-oriented programming (OOP) in Python
- Create classes to organize and structure data analysis code
- Implement methods to perform operations on data within class objects
Lesson 4: Working with Dates and Times in Python
- Use the
datetime
module to manipulate and analyze temporal data - Parse dates from strings using
strptime()
and format dates withstrftime()
- Perform calculations and comparisons with datetime objects
Guided Project: Exploring Hacker News Posts
- Apply data cleaning and analysis techniques to a real-world dataset
- Categorize and analyze posts based on their titles and creation times
- Extract insights about user engagement and posting patterns on Hacker News
Python Practice Problems
Test your knowledge with the Python exercises below. For additional practice problems and real-time feedback, try our interactive coding environment, great for Python practice online.
1. Helping Alice Compute Her GPA
In America, the metric that is used to evaluate a student's performance is the Grade Point Average (GPA). For the purpose of this problem, let's assume that the GPA of a student is calculated by carrying out the following sequence of steps:
- Multiply the individual grades of each course by the number of weekly hours and add them together.
- Calculate the total number of weekly hours.
- Divide the result from step 1 by the result of step 2.
For example, suppose that you have five courses — Mathematics, History, Science, Art, and English — and the following table gives your grades and course hours:
Course | Grade | Hours |
---|---|---|
Mathematics | 3 | 4 |
History | 3 | 2 |
Science | 4 | 3 |
Art | 2 | 2 |
English | 3 | 3 |
We can compute the GPA corresponding to the above table by following the three steps mentioned above.
- We multiply each grade by the number of hours and add them together:
$$ 3 \times 4 + 3 \times 2 + 4 \times 3 + 2 \times 2 + 3 \times 3 = 12 + 6 + 12 + 4 + 9 = 43 $$
- We add together the number of hours of each course:
$$ 4 + 2 + 3 + 2 + 3 = 14 $$
- We divide the result from the first step by the result from the second step:
$$ GPA = \frac{43}{14} \approx 3.07 $$
Instructions
- Create a variable
gpa
and assign it the value of Alice's GPA using the information contained in the following table (note that the table is different from the one above):
Course | Grade | Hours |
---|---|---|
Mathematics | 5 | 4 |
History | 2 | 2 |
Science | 5 | 3 |
Art | 3 | 2 |
English | 2 | 3 |
Hint
- Follow the three steps above.
- Compute $$ 5 \times 4 + 2 \times 2 + 5 \times 3 + 3 \times 2 + 2 \times 3 $$ and assign the result to a variable.
- Compute $$ 4 + 2 + 3 + 2 + 3 $$ and assign the result to a variable.
- Divide the first value by the second one.
Answer
step_1 = 5 * 4 + 2 * 2 + 5 * 3 + 3 * 2 + 2 * 3
step_2 = 4 + 2 + 3 + 2 + 3
gpa = step_1 / step_2
Practice solving this Python exercise using our interactive coding environment designed for Python practice online with real-time feedback. Try it here
2. Printing Stars
In this exercise, we'll ask you to create a long string programmatically, i.e., we expect you to build the required string using a small Python program and not by writing the string explicitly by hand.
Instructions
- Assign a string of length 128 that contains 128 times the character
*
to a variable namedstars
.
Hint
- Starting with
stars = '*'
, you can use the expressionstars += stars
a few times to achieve the goal.
Answer
# solution 1:
stars = '*'
stars += stars
stars += stars
stars += stars
stars += stars
stars += stars
stars += stars
stars += stars
# solution 2:
stars = '*' * 128
Practice solving this Python exercise using our interactive coding environment designed for Python practice online with real-time feedback.
3. Printing All Values
The list lines
contains the sentences of a poem. Your task is to print them to the screen.
lines = ["My candle burns at both ends;",
"It will not last the night;",
"But ah, my foes, and oh, my friends —",
"It gives a lovely light."]
Instructions
- Print all sentences contained in the
lines
list, one per line.
Hint
- Use a
for
loop and aprint()
statement inside it.
Answer
lines = ["My candle burns at both ends;",
"It will not last the night;",
"But ah, my foes, and oh, my friends —",
"It gives a lovely light."]
# Print each line
for line in lines:
print(line)
Practice solving this Python exercise using our interactive coding environment designed for Python practice online with real-time feedback.
4. Range For Loop
In this practice problem, you will have to print all numbers from 0 (inclusive) up to N (inclusive).
For this, we recommend that you use the range() built-in function.
You can use for var_name in range(2, 7):
to iterate a variable named var_name
over the numbers from 2 to 6. Note that the end of the range, 7, is exclusive. Therefore, the iteration will end at 6.
For example:
for i in range(2, 7):
print(i)
2
3
4
5
6
By default, the start of the range is 0. So if you only give one argument, it will be considered as the end of the range:
For example:
for i in range(7):
print(i)
0
1
2
3
4
5
6
Instructions
Assume the variable N = 11
has been initialized.
- Your task is to print all values from
0
toN
(inclusive).
Hint
- Use a
for
loop overrange(N + 1)
.
Answer
N = 11
# Print all values from 0 to N (inclusive)
for value in range(N + 1):
print(value)
Practice solving this Python exercise using our interactive coding environment designed for Python practice online with real-time feedback.
5. Understanding Function Scope 1
What will be the value of x
after executing the following code:
x = []
def f():
x = []
x.append(1)
x.append(2)
x.append(3)
return x
f()
print(x)
Instructions
- We propose a few possible answers:
- answer1 = None
- answer2 = []
- answer3 = [1, 2, 3]
Hint
- You can figure out the answer by running the code. But try to avoid it and understand why the answer is what it is.
Answer
answer1 = None
answer2 = []
answer3 = [1, 2, 3]
# Explanation:
"""
The variable x inside f() is not the same as x outside of it.
Therefore, x outside the function never gets modified when we call f().
"""
correct = answer2
Practice solving this Python exercise using our interactive coding environment designed for Python practice online with real-time feedback.
6. Understanding Function Scope 2
What will be the value of x
after executing the following code:
x = []
def f():
x = []
x.append(1)
x.append(2)
x.append(3)
f()
print(x)
Instructions
- We propose a few possible answers:
- answer1 = None
- answer2 = []
- answer3 = [1, 2, 3]
Hint
- You can figure out the answer by running the code. But try to avoid it and understand why the answer is what it is.
Answer
answer1 = None
answer2 = []
answer3 = [1, 2, 3]
# Explanation:
"""
The variable x inside f() is not the same as x outside of it.
Therefore, x outside the function never gets modified when we call f().
"""
correct = answer2
Practice solving this Python exercise using our interactive coding environment designed for Python practice online with real-time feedback.
7. Person Class
In this practice problem, you'll create a class to represent a person. For simplicity, we will only store the first and last names of a person.
We want you to implement a class named Person
with the following methods:
__init__(self, first_name, last_name)
: This method creates aPerson
instance by storingfirst_name
intoself.first_name
andlast_name
intoself.last_name
.__str__(self)
: This method computes a string representation of this person. The format of this string should be the first name, followed by a space, and then the last name. In each name, we want all characters to be in lower case, except for the first one that should be in upper case.
Examples of usage:
person = Person('bruno', 'LopeZ')
print(person)
Bruno Lopez
person = Person('aNNa', 'martin')
print(person)
Anna Martin
Instructions
- Define a class named
Person
. - Define the
__init__()
method with three arguments:self
: The self-reference of the class instance.first_name
: The first name of the person.last_name
: The last name of the person.
- Implement the
__init__()
method so that it storesfirst_name
inself.first_name
andlast_name
inself.last_name
. - Define a
__str__()
method with one argument:self
: The self-reference of the class instance.
- Implement the
__str__()
method so that it returns a string representation of this person. The format of this string should be the first name, followed by a space, and then the last name. In each name, we want all characters to be in lower case, except for the first one that should be in upper case.
Optional steps to test your solution:
- Create an instance of
Person
using"EmiLia"
for the first name and"GomEZ"
as the last name and assign it to a variable namedperson
. - Print the value of
person
. The result should beEmilia Gomez
.
Hint
- To make all characters of a string uppercase, you can use the
str.upper()
method. - In the same way, to make all characters in a string lowercase, you can use the
str.lower()
method.
Answer
class Person:
def __init__(self, first_name, last_name):
self.first_name = first_name[0].upper() + first_name[1:].lower()
self.last_name = last_name[0].upper() + last_name[1:].lower()
def __str__(self):
return '{} {}'.format(self.first_name, self.last_name)
# Example usage
person = Person("EmiLia", "GomEZ")
print(person)
Practice solving this Python exercise using our interactive coding environment designed for Python practice online with real-time feedback.
8. 2D Points
In this practice problem, you'll create a class to represent points in two dimensions. A point in two dimensions is essentially a pair of numbers.
The following figure shows points (0, 0), (2, 4) and (4, 1):
We want you to implement a class named Point2D
with the following methods:
__init__(self, x, y)
: This method creates aPoint2D
instance by storingx
intoself.x
andy
intoself.y
.distance(self, other)
: This method computes the distance between pointsself
andother
.
The distance between two points (x1, y1) and (x2, y2) is calculated with the following formula:
\[ \sqrt{(x_1 - x_2)^2 + (y_1 - y_2)^2} \]
For example, the distance between points (2, 4) and (4, 1) is approximately equal to 3.605551, as shown in the figure:
In Python, you can compute the square root of a number by using the math.sqrt() function from the math
module. Here are a few examples:
import math
print(math.sqrt(9))
print(math.sqrt(1))
print(math.sqrt(42))
3.0
1.0
6.48074069840786
Here is an example of how someone might use your class once implemented:
point1 = Point2D(2, 4)
point2 = Point2D(4, 1)
distance = point1.calculate_distance(point2)
print(distance)
3.605551275463989
Instructions
- Define a class named
Point2D
. - Define the
__init__()
method with three arguments:
self
: The self-reference of the class instance.x
: The value of the x-coordinate.y
: The value of the y-coordinate.
__init__()
method so that it stores x
in self.x
and y
in self.y
.calculate_distance()
method with two arguments:self
: The self-reference of the class instance.other
: Another instance ofPoint2D
to which we want to compute the distance.
calculate_distance()
method so that it returns the distance between this point and the one given as argument.Optional steps to test your solution:
- Create an instance of
Point2D
to represent point (3, 4) and assign it to variablepoint1
. - Create an instance of
Point2D
to represent point (9, 5) and assign it to variablepoint2
. - Test your class by calculating the distance between
point1
andpoint2
and assign the result to a variable nameddistance
. - Print the value of
distance
. The result should be approximately equal to6.082762530298219
.
Hint
- Remember to pass the
self
argument as the first argument of both methods. - Remember to import the
math
module and use themath.sqrt()
function to compute square roots.
Answer
import math
class Point2D:
def __init__(self, x, y):
self.x = x
self.y = y
def calculate_distance(self, other):
dx = self.x - other.x
dy = self.y - other.y
return math.sqrt(dx * dx + dy * dy)
# Example usage
point1 = Point2D(3, 4)
point2 = Point2D(9, 5)
distance = point1.calculate_distance(point2)
print(distance)
Practice solving this Python exercise using our interactive coding environment designed for Python practice online with real-time feedback.
Python Cheat Sheet
Check out our comprehensive Python Cheat Sheet that provides a quick reference for essential Python commands.
You can also download the Python Cheat Sheet as a PDF.
Python Practice
The best way to get Python practice is to work on a real world challenge in the form of projects. Use these Dataquest guided projects to test your skills and show off your knowledge to potential employeers by including them in your portfolio.
1. Profitable App Profiles for the App Store and Google Play Markets
Difficulty Level: Beginner
Overview
In this beginner-level guided project, you'll step into the role of a data scientist for a company that builds ad-supported mobile apps. Using Python and Jupyter Notebook, you'll analyze real datasets from the Apple App Store and Google Play Store to identify app profiles that attract the most users and generate the highest revenue. By applying data cleaning techniques, conducting exploratory data analysis, and making data-driven recommendations, you'll develop practical skills essential for entry-level data science positions.
Tools and Technologies
- Python
- Jupyter Notebook
Prerequisites
To successfully complete this project, you should be comfortable with Python fundamentals such as:
- Variables, data types, lists, and dictionaries
- Writing functions with arguments, return statements, and control flow
- Using conditional logic and loops for data manipulation
- Working with Jupyter Notebook to write, run, and document code
Step-by-Step Instructions
- Open and explore the App Store and Google Play datasets
- Clean the datasets by removing non-English apps and duplicate entries
- Analyze app genres and categories using frequency tables
- Identify app profiles that attract the most users
- Develop data-driven recommendations for the company's next app development project
Expected Outcomes
Upon completing this project, you'll have gained valuable skills and experience, including:
- Cleaning and preparing real-world datasets for analysis using Python
- Conducting exploratory data analysis to identify trends in app markets
- Applying frequency analysis to derive insights from data
- Translating data findings into actionable business recommendations
Relevant Links and Resources
2. Exploring Hacker News Posts
Difficulty Level: Beginner
Overview
In this beginner-level guided project, you'll analyze a dataset of submissions to Hacker News, a popular technology-focused news aggregator. Using Python and Jupyter Notebook, you'll explore patterns in post creation times, compare engagement levels between different post types, and identify the best times to post for maximum comments. This project will strengthen your skills in data manipulation, analysis, and interpretation, providing valuable experience for aspiring data scientists.
Tools and Technologies
- Python
- Jupyter Notebook
Prerequisites
To successfully complete this project, you should be comfortable with Python concepts for data science such as:
- String manipulation and basic text processing
- Working with dates and times using the datetime module
- Using loops to iterate through data collections
- Basic data analysis techniques like calculating averages and sorting
- Creating and manipulating lists and dictionaries
Step-by-Step Instructions
- Load and explore the Hacker News dataset, focusing on post titles and creation times
- Separate and analyze 'Ask HN' and 'Show HN' posts
- Calculate and compare the average number of comments for different post types
- Determine the relationship between post creation time and comment activity
- Identify the optimal times to post for maximum engagement
Expected Outcomes
Upon completing this project, you'll have gained valuable skills and experience, including:
- Manipulating strings and datetime objects in Python for data analysis
- Calculating and interpreting averages to compare dataset subgroups
- Identifying time-based patterns in user engagement data
- Translating data insights into practical posting strategies
Relevant Links and Resources
Python Frequently Asked Questions
What is Python and why is it popular for data science?
Python is a versatile and easy-to-use programming language that has become a favorite among data scientists. Its simplicity and readability make it an ideal choice for beginners and experts alike.
So, what makes Python so popular in data science? Here are a few key reasons:
- Python has a wide range of libraries that make data analysis and visualization easy. For example,
pandas
is a library that helps you work with data, whilematplotlib
is a library that helps you create visualizations. - Python is great for handling large datasets efficiently.
- Python has extensive machine learning capabilities, which is essential for data science tasks like building predictive models.
In data science, Python is commonly used for tasks like data cleaning, exploratory analysis, and building machine learning models. For instance, you might use Python to analyze data from an app store, identifying popular app categories and trends. Or you could use it to explore patterns in user engagement on social media platforms, determining the best times to post for maximum engagement.
If you're new to Python, don't worry! We have many tutorials and resources available to help you get started. These tutorials include hands-on projects that allow you to apply your skills to real-world data problems, such as analyzing mobile app markets or exploring social media post engagement.
By learning Python, you'll be well-equipped to tackle a wide range of data challenges and uncover valuable insights from complex datasets. With its ease-of-use and powerful capabilities, Python is an essential tool in any data scientist's toolkit.
What are the essential components of a comprehensive Python for data science tutorial?
A comprehensive Python tutorial for data science should cover several key components. Fundamental Python concepts form the foundation, including variables, data types, loops, and functions. The tutorial should emphasize data structures like lists and dictionaries, which are essential for organizing and manipulating data efficiently.
For data science applications, the Python tutorial must introduce specialized libraries such as numpy
, pandas
, and matplotlib
. These tools enable efficient data manipulation, analysis, and visualization. Additionally, learning to work with Jupyter Notebook is vital, as it provides an interactive environment for coding and documenting data science workflows.
Hands-on practice is a key element of any effective Python tutorial. This includes coding exercises that reinforce basic concepts, as well as more complex projects that simulate real-world data challenges. For example, analyzing app store data or exploring user engagement patterns can provide practical experience in data cleaning, exploratory analysis, and deriving insights.
As you progress, advanced topics in a Python tutorial might cover data cleaning techniques, exploratory data analysis, and an introduction to machine learning concepts. Understanding function scope and debugging techniques is also important for writing efficient and error-free code.
Supplementary resources like cheat sheets and FAQs can enhance the learning experience, providing quick references for syntax and common operations. By combining theoretical knowledge with practical application, a comprehensive Python tutorial equips aspiring data scientists with the skills needed to tackle complex data challenges confidently.
How does Python compare to SQL for data analysis tasks?
Python and SQL are both essential tools in a data analyst's toolkit, each with its own strengths. When it comes to data manipulation, analysis, and visualization, Python is a powerful and versatile language. Its extensive libraries, such as pandas and NumPy, make it easy to efficiently clean, transform, and analyze complex datasets.
On the other hand, SQL is ideal for working with relational databases. It excels at querying large datasets, performing aggregations, and joining tables. SQL is particularly useful when you need to extract specific data from structured databases.
In many projects, I find myself using both languages together. For instance, I might use SQL to pull relevant data from a database, and then switch to Python for more advanced analysis or to create visualizations. By combining the strengths of both languages, I can tackle a wide range of data challenges.
So, how do you decide which language to use? It really depends on the task at hand. Here are some general guidelines:
I use Python for tasks such as:
- Cleaning and preprocessing unstructured data
- Performing complex statistical analyses
- Creating data visualizations
- Building machine learning models
I use SQL for tasks such as:
- Querying large relational databases
- Performing aggregations on structured data
- Joining data from multiple tables
In my experience, having a good understanding of both Python and SQL is essential for effective data analysis. That's why I always try to include sections on integrating SQL queries into Python code in my tutorials. By learning both languages, you'll be well-equipped to handle diverse data analysis tasks and extract valuable insights from any dataset you encounter.
What types of Python practice problems are most beneficial for aspiring data scientists?
For aspiring data scientists, the most beneficial Python practice problems are those that build a strong foundation in programming basics while developing applied data analysis skills. As you work through a Python tutorial, it's essential to tackle a variety of problem types.
Programming Fundamentals: Start with problems that reinforce basic concepts. For example, calculating a student's GPA using given grades and course hours helps solidify your understanding of variables, data types, and arithmetic operations in Python. These fundamentals are the building blocks for more complex data analysis tasks.
Data Structures: Becoming comfortable with lists and dictionaries is essential for data science. Practice problems like creating a string of 128 asterisks or generating frequency tables from datasets help you develop these skills. These skills directly translate to handling real-world datasets in your future projects.
Control Flow and Functions: Writing functions and using loops are key skills for efficient data processing. Try creating functions to explore datasets or calculate frequency tables. These exercises develop your ability to structure code and handle repetitive tasks - skills you'll use daily as a data scientist.
Applied Data Analysis: As you progress, focus on problems that simulate real-world data tasks. Analyzing app store data or exploring patterns in social media engagement allows you to apply multiple concepts to practical scenarios. These problems help bridge the gap between basic Python skills and actual data science work.
The guided projects in our comprehensive Python tutorial provide excellent opportunities to apply these skills to realistic challenges. For example, analyzing app profiles for marketplaces or exploring Hacker News posts combines multiple concepts and requires critical thinking about data cleaning, analysis, and interpretation.
When tackling practice problems, start with simpler tasks and gradually increase complexity. For fundamental concepts, focus on understanding the logic behind your solutions. As you move to more complex problems, pay attention to code efficiency and reusability. Don't hesitate to revisit and refine your solutions as you learn new techniques.
Consistent practice across various problem types is key to developing your Python skills for data science applications. By regularly challenging yourself with diverse problems, you'll build the skills and confidence needed to tackle real-world data challenges in your future career.
How can I use Jupyter Notebook to enhance my Python learning experience?
Jupyter Notebook is an interactive computing environment that can greatly enhance your experience when working through a Python tutorial. By allowing you to write and execute code in small chunks, you can see the results immediately, which is especially helpful for beginners. This interactivity enables you to experiment with Python concepts and get instant feedback, making it easier to learn and understand.
One of the key benefits of using Jupyter Notebook for Python learning is the ability to combine code, outputs, and explanatory text in a single document. For example, when learning about functions, you can define a function in one cell, test it in another, and add notes about how it works in between. This approach makes it easier to document your learning process and create comprehensive, shareable notebooks of your progress through Python tutorials.
Additionally, Jupyter Notebook offers special features that can aid your learning. For instance, you can use magic commands like %timeit
to measure the execution time of your code, helping you understand performance implications as you learn. Whether you're just starting with Python or advancing your skills, Jupyter Notebook's flexibility and ease of use make it a valuable tool for working through Python tutorials and building a strong foundation in programming.
What are some practical Python projects for beginners in data science?
As you learn Python, it's essential to apply your skills to real-world projects. This helps you build confidence in your coding abilities and develop a portfolio to showcase your skills. So, what kinds of projects should you tackle as a beginner in data science?
One great project to start with is our guided project on analyzing app store data to identify profitable app profiles. This project allows you to practice working with lists, dictionaries, and functions while conducting frequency analysis on real datasets. You'll also get to apply skills like data cleaning, exploratory analysis, and deriving actionable insights.
Another valuable project is is our guided project on exploring patterns in social media post engagement by analyzing Hacker News submissions. This helps you understand how to work with dates and times, manipulate strings, and perform basic statistical analysis in Python. Both projects simulate real-world data science tasks, making it easier to apply concepts from Python tutorials to practical challenges.
By working on these projects, you'll gain hands-on experience and develop a portfolio that showcases your skills. This experience will prepare you for more advanced projects and potential career opportunities in the field.
What are the key Python concepts covered in an introductory Python tutorial?
When you explore a Python tutorial, you'll encounter several key concepts that form the foundation of programming and data analysis. These essential building blocks will give you the skills to write efficient code and tackle real-world data challenges.
Let's break down the essential Python concepts you'll typically learn:
- Python basics:
- Variables and data types (integers, floats, strings)
- Basic operators for arithmetic and comparisons
- Lists and dictionaries for organizing data
- Control flow:
- For loops for iterating through data
- Conditional statements (if/else) for decision-making
- Functions for organizing and reusing code
- Data manipulation:
- String manipulation and text processing
- Working with dates and times
- Basic data analysis techniques like calculating averages
- Programming tools:
- Using Jupyter Notebook for interactive coding
- Importing and working with modules like datetime
These foundational elements allow you to store, manipulate, and organize information. For example, you might use a list to store a series of stock prices or a dictionary to map product names to their sales figures.
These concepts give your code the ability to make decisions and repeat tasks efficiently. For instance, you might use a for loop to calculate the average rating for thousands of mobile apps, or create a function that cleans and preprocesses raw data from multiple sources.
These skills are essential for preparing and analyzing data. You might apply string manipulation to extract relevant information from user comments, or use date/time functions to identify trends in website traffic over time.
These tools enhance your coding experience and extend Python's capabilities. Jupyter Notebook, for example, allows you to write, run, and document your code in a single interactive environment – perfect for data exploration and analysis.
As you progress through our Python tutorial, you'll see how these concepts come together to solve complex problems. For example, you see how to combine loops, conditional statements, and string manipulation to analyze thousands of social media posts, categorizing them based on content and sentiment.
Developing a strong foundation in these fundamental concepts will serve as a stepping stone to more advanced Python programming and data science techniques. Whether you're aiming to build machine learning models, create data visualizations, or automate data processing tasks, these core skills will help you succeed in the world of Python and data analysis.
How can I practice Python online with real-time feedback?
Practicing Python online with real-time feedback is a great way to improve your coding skills. This approach allows you to get immediate responses to your code, which helps you learn and correct mistakes more efficiently.
Here are some effective ways to practice Python online and get real-time feedback:
-
Try interactive coding environments: Platforms like Jupyter Notebook, which we use in our Python tutorials, let you write and execute code in small chunks. This is especially helpful for beginners, as it provides instant results and helps you see how different parts of your code work together.
-
Work on guided projects: Our Python tutorial includes projects like "Profitable App Profiles for the App Store and Google Play Markets" and "Exploring Hacker News Posts". These projects give you real-world scenarios to apply your Python skills and get immediate feedback on your approach.
-
Use online practice problems: Our interactive Python coding environment offers a range of Python practice problems with real-time feedback. These exercises cover various difficulty levels and concepts, helping you reinforce your learning from the Python tutorial.
-
Experiment with different data structures: As you learn about lists, dictionaries, and other data structures in the Python tutorial, try manipulating them in an interactive environment. This hands-on practice helps solidify your understanding of how these structures work.
By using these online resources and taking advantage of real-time feedback, you'll be able to practice Python more effectively and build your skills faster. Remember, consistent practice and applying concepts from your Python tutorial are key to becoming proficient in the language. Don't be afraid to try new things and learn from both your successes and mistakes in these interactive environments.
What are some common challenges faced by beginners when learning Python for data science?
Learning Python for data science can be challenging, but understanding common pitfalls can help you overcome them. Here are some key areas to focus on:
-
Getting to grips with core programming concepts: Ideas like variables, functions, and control flow may seem abstract at first. Think of it like learning a new language – the more you practice, the more comfortable you'll become. Try writing small programs to solidify these concepts.
-
Working with data structures: Lists and dictionaries are essential in data science, but can be confusing initially. Experiment with creating and manipulating these structures in interactive Python environments to build familiarity. It's like learning to juggle – it takes time and practice to get the hang of it.
-
Applying Python to real-world data: Bridging the gap between basic syntax and actual data analysis can be tricky. Start with small datasets, like analyzing app store ratings, and gradually work up to more complex problems. This will help you build confidence and develop a deeper understanding of how to apply Python to real-world problems.
-
Debugging and troubleshooting: Identifying and fixing errors in your code is an essential skill. Learn to read error messages and use print statements to understand what's happening in your program. It's like being a detective – you need to gather clues to solve the mystery.
Remember, hands-on practice is essential. Work through Python tutorials, tackle practice problems, and apply your skills to real-world datasets. By overcoming these challenges, you'll develop valuable problem-solving skills and gain the ability to extract meaningful insights from data.
Don't be discouraged by initial difficulties – every data scientist started as a beginner. With persistence and consistent practice, you'll become proficient in Python and open up exciting opportunities in the world of data science.
How can developing Python skills create opportunities in advanced data analysis and machine learning?
When I first started learning Python, I had no idea how it would transform my approach to data analysis and open doors to exciting opportunities in machine learning and AI. Now, as someone who oversees Python course development, I can confidently say that developing Python skills is one of the best investments you can make for your data career.
Python's versatility is what makes it so powerful for advanced data analysis and machine learning. As you progress from basic concepts like variables and lists to more complex operations, you'll find that Python becomes an indispensable tool in your data toolkit. For instance, I recently used Python to automate our course prerequisite writing process, a task that would have been challenging with SQL alone.
As you gain more experience with Python, you'll discover its true potential in advanced data analysis. Libraries like pandas and NumPy allow you to manipulate and analyze large datasets efficiently. You can create insightful visualizations with matplotlib, perform statistical analyses with SciPy, and even build machine learning models with scikit-learn.
The journey from basic Python skills to advanced applications is a natural progression. You start with simple operations like calculating averages or filtering data, and before you know it, you're implementing complex algorithms and building predictive models. For example, at Dataquest, we use Python to analyze student progress data, calculate course completion rates, and identify areas for improvement in our curriculum.
What's particularly exciting about Python is its widespread use in the field of machine learning and AI. As you become more proficient, you'll be able to tackle tasks like natural language processing with Python, image recognition, and predictive modeling. These skills are in high demand across industries, from tech companies building recommendation systems to healthcare organizations developing diagnostic tools.
With each new concept you learn, you're building a foundation for more advanced applications. Whether you're analyzing customer behavior, optimizing business processes, or developing cutting-edge AI applications, Python provides the tools and flexibility to bring your ideas to life.
By investing in your Python skills, you're not just learning a programming language – you're opening doors to exciting career opportunities in data science, machine learning engineering, and AI research. And the best part? Python is accessible to anyone willing to learn, regardless of their background. So why wait? Start your Python journey today and see where it takes you in the world of advanced data analysis and machine learning.