Path overview
In this path, you’ll become familiar with SQL syntax and master the frequently used commands. You’ll also learn how to use string patterns and ranges to query data, how to sort and group data, and how to write perfect queries to extract and analyze data from real SQL databases.
Best of all, you’ll learn by doing – you’ll write code and get feedback directly in the browser. You’ll apply your skills to several guided projects involving realistic business scenarios to build your portfolio and prepare for your next interview.
Key skills
- Exploring, querying, and extracting specific sets of data
- Handling data from multiple sources
- Retrieving data to build reports and perform analysis
- Organizing data efficiently to answer business questions
Path outline
Part 1: Fundamentals of SQL [5 courses]
Introduction to SQL and Databases 5h
Objectives- Define the structure of SQL
- Create basic queries to extract data from tables in a database
- Define databases
- Identify different versions of SQL
- Write good SQL code
Summarizing Data in SQL 3h
Objectives- Employ SQL to compute statistics
- Provide statistics by group
- Filter results over groups
Combining Tables in SQL 4h
Objectives- Combine tables using inner joins
- Employ different types of joins
- Employ other SQL clauses with joins
- Join on complex conditions
- Employ set operators like UNION and EXCEPT
SQL Subqueries 6h
Objectives- Nest a query inside another query
- Employ different types of subqueries
- Employ common table expressions
- Scale your project with complex queries
Window Functions in SQL 7h
Objectives- Set up a frame for window functions
- Compute running aggregations with aggregate window functions
- Explore rank window functions
- Apply distribution window functions
- Use offset window functions
The Dataquest guarantee
Dataquest has helped thousands of people start new careers in data. If you put in the work and follow our path, you’ll master data skills and grow your career.
We believe so strongly in our paths that we offer a full satisfaction guarantee. If you complete a career path on Dataquest and aren’t satisfied with your outcome, we’ll give you a refund.
Master skills faster with Dataquest
Go from zero to job-ready
Learn exactly what you need to achieve your goal. Don’t waste time on unrelated lessons.
Build your project portfolio
Build confidence with our in-depth projects, and show off your data skills.
Challenge yourself with exercises
Work with real data from day one with interactive lessons and hands-on exercises.
Showcase your path certification
Share the evidence of your hard work with your network and potential employers.
Projects in this path
Analyzing Kickstarter Projects
For this project, you’ll assume the role of a data analyst at a startup considering launching a Kickstarter campaign. You’ll analyze data to help the team understand what might influence a campaign’s success.
Customers and Products Analysis Using SQL
For this project, you’ll step into the role of a data analyst at a scale model car company. You’ll use SQL skills like joins and subqueries to explore a sales database and provide data-driven answers to key business questions about inventory, customers, and marketing.
SQL Window Functions for Northwind Traders
For this project, you’ll assume the role of a data analyst at Northwind Traders, using advanced SQL techniques to provide insights that drive strategic business decisions.
Learning resources
Grow your career with
Dataquest.
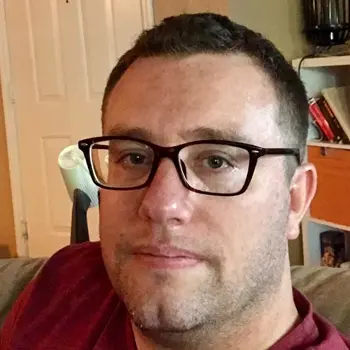
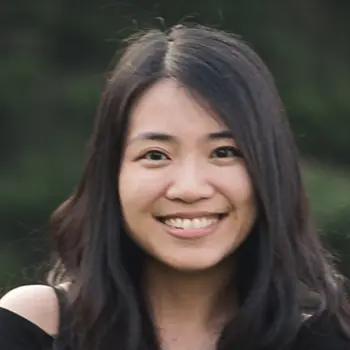
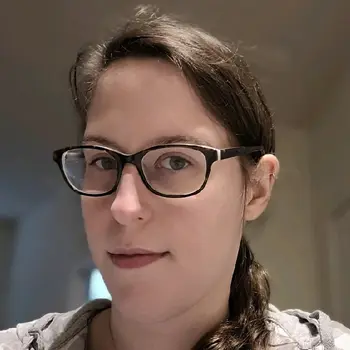